How to map a Java Bean using mapstruct library ?
in cs
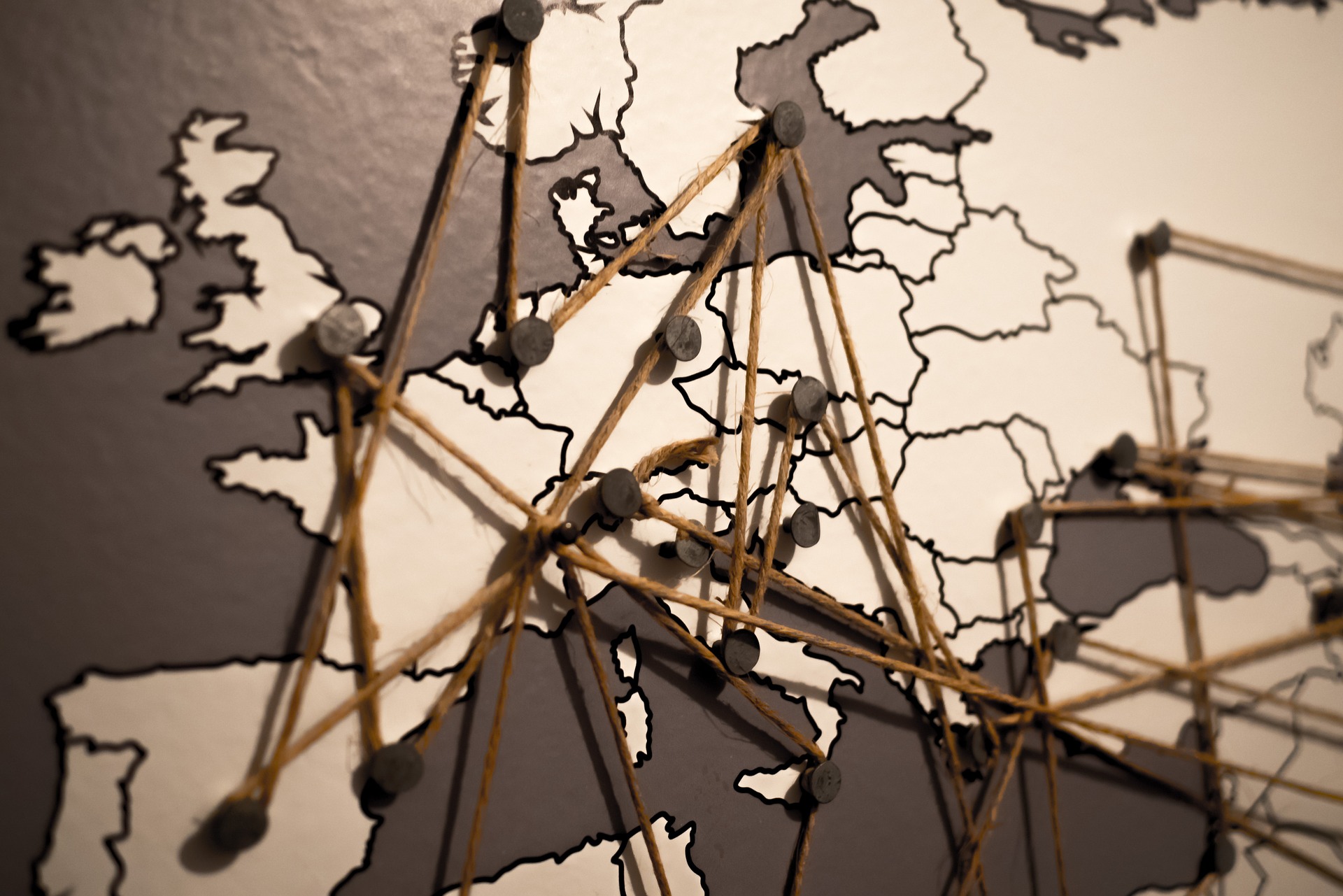
Five min introduction on mapstruct for Java Bean Mapping.
- Why mapping ?
- Example Project Setup
- How to map using mapstruct annotation ?
- Testing
- How it works ?
- References
- Conclusion
This blog, I am going to show a simple example for the understanding on Java Bean Mapping.
Why mapping ?
- Convert Java Bean/POJO to Another Bean/POJO with different names.
- Create Report Json with additional calculations.
- For using DTO Design Pattern.
Example Project Setup
git clone https://github.com/notesbyair/blog.mapstruct.git
In this example, we have a Java Bean - Car
, we want to convert it to `CarDTO object where it is has different names for mapping.
How to map using mapstruct annotation ?
Define a mapper interface for above conversion, it will convert Car
to CarDto
. The mapstruct library generates the implementations for it.
In below interface source:Car
, target: CarDto
.
// file: "CarMapper.java"
import org.mapstruct.Mapper;
import org.mapstruct.Mapping;
import org.mapstruct.factory.Mappers;
@Mapper
public interface CarMapper {
CarMapper INSTANCE = Mappers.getMapper( CarMapper.class );
@Mapping(target = "seats", source = "seatConfiguration")
CarDto convert(Car car);
@Mapping(source = "numberOfSeats", target = "seatCount")
@Mapping(source = "seatMaterial", target = "material")
SeatConfigurationDto seatConfigurationToSeatConfigurationDto(SeatConfiguration seatConfiguration);
}
CarMapper for Converting Car to CarDTO
Testing
@Test
void testMapping() {
Car car = new Car();
car.setMake("Tesla");
car.setType(CarType.SPORTS);
car.setSeatConfiguration(new SeatConfiguration(1, SeatMaterial.LEATHER));
CarDto carDTO = CarMapper.INSTANCE.convert(car);
assertEquals(car.getMake(), carDTO.getMake());
assertEquals(car.getType().toString(), carDTO.getType());
assertEquals(car.getSeatConfiguration().getSeatMaterial().toString(),
carDTO.getSeats().getMaterial());
}
How it works ?
- If Source and Target bean contains same name, then it will convert directly, for example
make
is same name in both beans. For all other, you need to define a names usingmapping
annotation like shown in above. - You need to define another mapping, which will convert the
seatConfiguration
. For more, refer this section of the blog post
References
Conclusion
Using mapstruct
, we can easily map Java Beans/POJO using less number of codes. Hope this gives nice introduction, let me know for any doubts here.